LaravelからContentfulの記事を取得する方法についてメモ。
Contentful PHP SDKをインストール。
composer require contentful/rich-text
サービスプロバイダーに追加。config/app.phpの中に1行追記。
return [
'providers' => [
// ・・・・・
Contentful\Laravel\ContentfulServiceProvider::class, // 追記
// ・・・・・
]
];
Contentful SDK用の設定ファイルを作成するコマンド実行
php artisan vendor:publish --provider="Contentful\Laravel\ContentfulServiceProvider"
config/contentful.phpが作られる。
ここは触らなくてOK。
<?php
/**
* This file is part of the contentful/laravel package.
*
* @copyright 2015-2020 Contentful GmbH
* @license MIT
*/
declare(strict_types=1);
return [
'delivery' => [
/*
* The ID of the space you want to access.
*/
'space' => env('CONTENTFUL_SPACE_ID'),
/*
* The ID of the environment you want to access.
*/
'environment' => env('CONTENTFUL_ENVIRONMENT_ID', 'master'),
/*
* An API key for the above specified space.
*/
'token' => env('CONTENTFUL_DELIVERY_TOKEN'),
/*
* Controls whether Contentful's Delivery or Preview API is accessed.
*/
'preview' => (bool) env('CONTENTFUL_USE_PREVIEW', false),
/*
* The default locale to use when querying the API.
*/
'defaultLocale' => env('CONTENTFUL_DEFAULT_LOCALE'),
/*
* An array of further client options. See Contentful\Delivery\Client::__construct() for more.
*/
'delivery.options' => [],
],
];
envファイルに追記
CONTENTFUL_SPACE_IDと、CONTENTFUL_DELIVERY_TOKENと、CONTENTFUL_ENVIRONMENT_IDを追記。
これらの情報に関しては、Contentfulにログインして、取得したい記事のあるスペースを選択、Settings -> API keys から確認できる。APIキーをまだ作ってないときは、Example Key1を見ながら作る。
// .envファイル
CONTENTFUL_SPACE_ID={Space IDをコピペ}
CONTENTFUL_DELIVERY_TOKEN={Content Delivery API - access tokenをコピペ}
CONTENTFUL_ENVIRONMENT_ID={Environmentsの欄を見て設定してる環境を書く。masterとか}
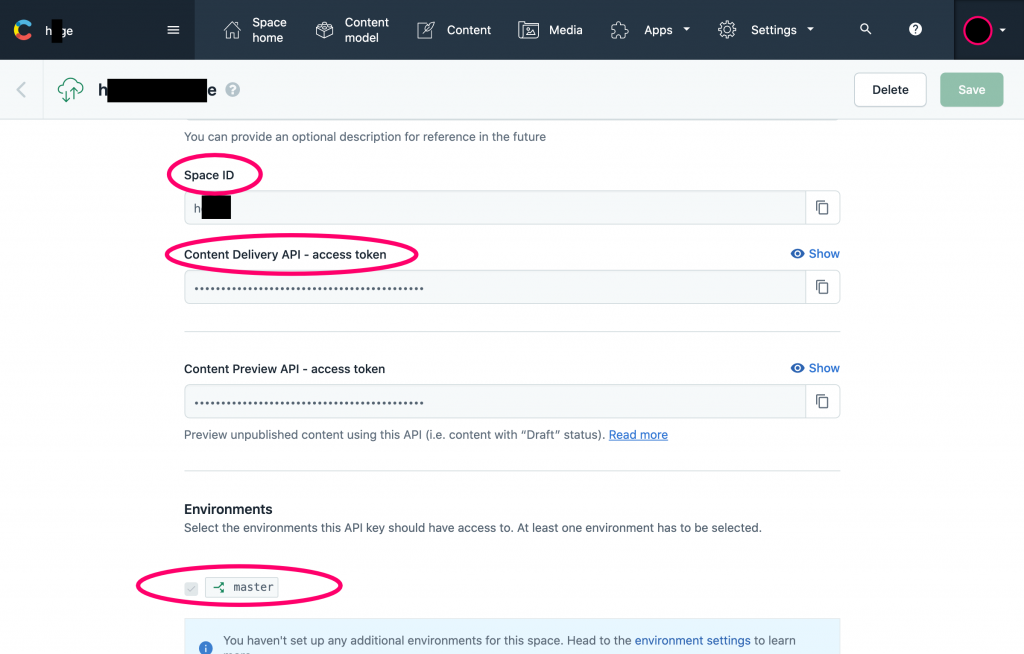
ここまでで、Laravelでcontentfulを使う準備はOK。
controller用意
Contentful SDKを使って、API叩けばデータが帰ってくる。
<?php
namespace App\Http\Controllers\Api;
use Illuminate\Routing\Controller as BaseController;
class HogeController extends BaseController
{
/**
* Create a new controller instance.
*
* @return void
*/
public function index()
{
$query = new \Contentful\Delivery\Query();
$query->setContentType('post')
->orderBy('-sys.createdAt')
->setLimit(3);
$entries = $this->client->getEntries($query);
$posts = [];
foreach ($entries as $entry) {
$posts[] = [
'entry_id' => $entry->getId(),
'release_date' => date('Y.m.d', strtotime($entry->get('release_date'))),
'title' => $entry->get('title'),
'category' => $entry->get('category')->get('name')
];
}
return $posts;
}
}
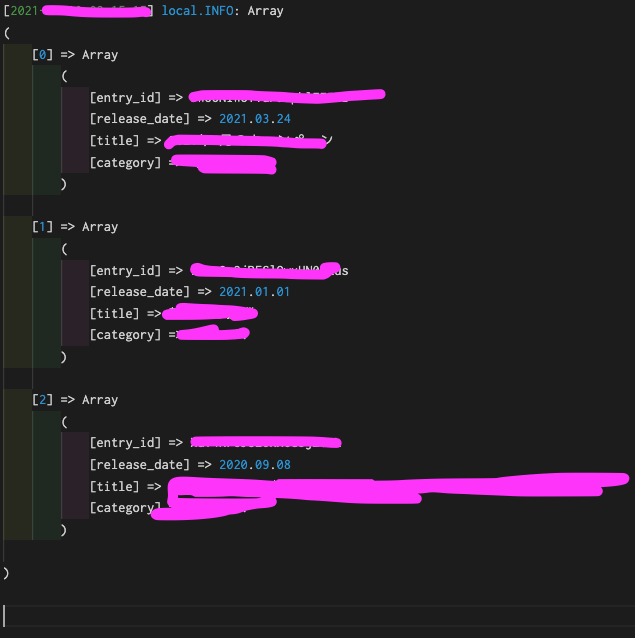
ログに出したらContentfulからデータが取れていた。